You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Hey, I have the following issue where:
the color is switched
so RGBA(255, 0, 255, 255) (Pink, 100% Alpha)
turns into yellow and
RGBA(255, 255, 0, 255) (Yellow, 100% Alpha)
turns into pink
Color class is just a helper class to use RGB values instead of hex values.
But to prove it's not the fault of the class, here is an example using hex values:
Yeah, I think I just noticed roughly the same issue just now. The problem here is endianness. The upper 8 bits are the alpha, followed by blue, then green, then red - in essence, this is ABGR in memory. See Cherno's Raytracing code to see what I mean.
Hey, I have the following issue where:
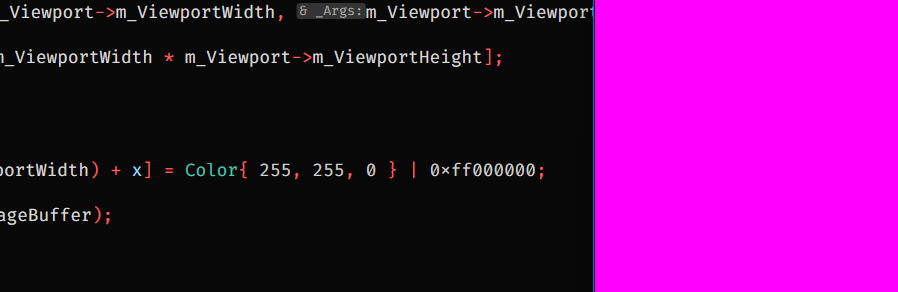
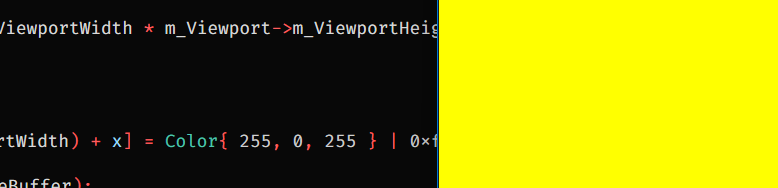
the color is switched
so RGBA(255, 0, 255, 255) (Pink, 100% Alpha)
turns into yellow and
RGBA(255, 255, 0, 255) (Yellow, 100% Alpha)
turns into pink
my app code is pretty simple:
The text was updated successfully, but these errors were encountered: